Understanding the data structure#
In this chapter, you will learn about the data structure of n8n and how to use the Code node to transform data and simulate node outputs.
Data structure of n8n#
In a basic sense, n8n nodes function as an Extract, Transform, Load (ETL) tool. The nodes allow you to access (extract) data from multiple disparate sources, modify (transform) that data in a particular way, and pass (load) it along to where it needs to be.
The data that moves along from node to node in your workflow must be in a format (structure) that can be recognized and interpreted by each node. In n8n, this required structure is an array of objects.
About array of objects
An array is a list of values. The array can be empty or contain several elements. Each element is stored at a position (index) in the list, starting at 0, and can be referenced by the index number. For example, in the array ["Leonardo", "Michelangelo", "Donatello", "Raphael"];
the element Donatello
is stored at index 2.
An object stores key-value pairs, instead of values at numbered indexes as in arrays. The order of the pairs isn't important, as the values can be accessed by referencing the key name. For example, the object below contains two properties (name
and color
):
1 2 3 4 |
|
An array of objects is an array that contains one or more objects. For example, the array turtles
below contains four objects:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
You can access the properties of an object using dot notation with the syntax object.property
. For example, turtles[1].color
gets the color of the second turtle.
Data sent from one node to another is sent as an array of JSON objects. The elements in this collection are called items.
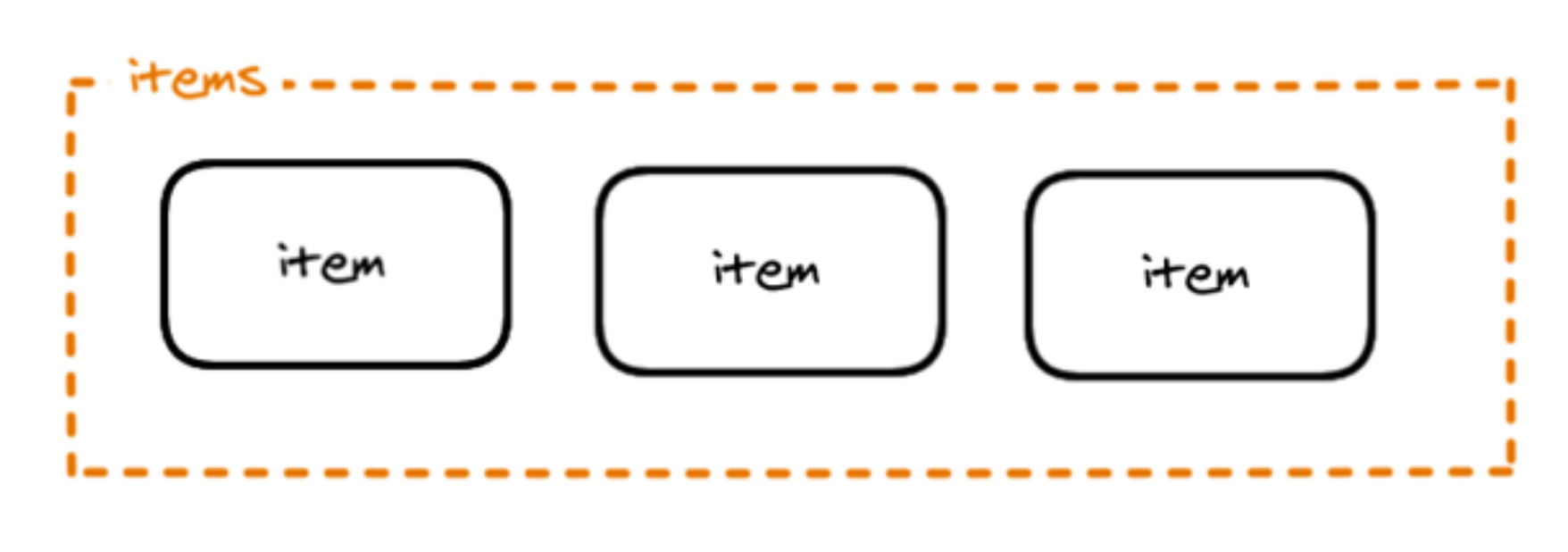
An n8n node performs its action on each item of incoming data.
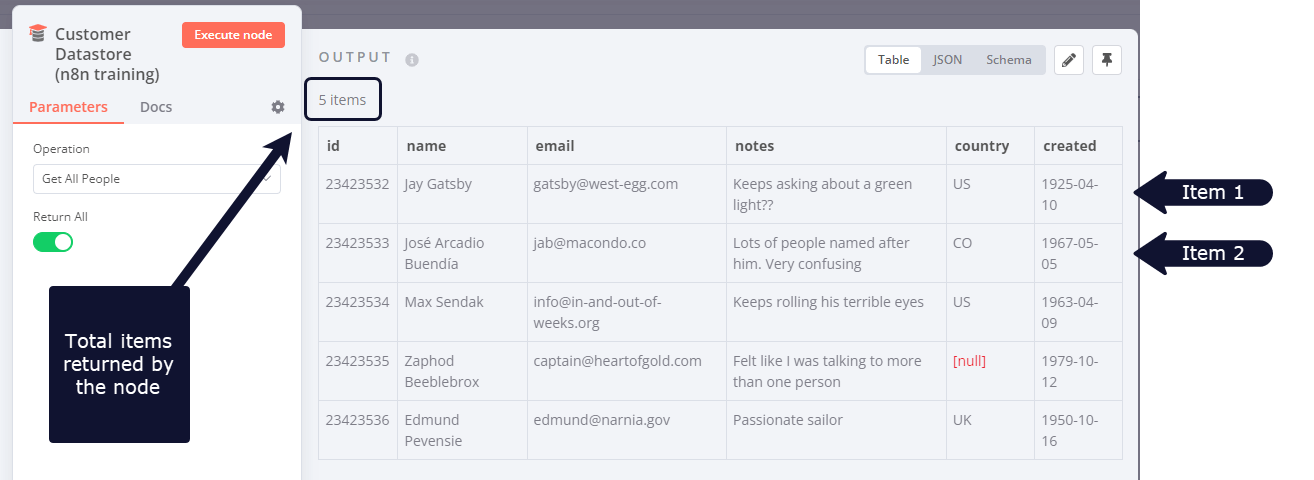
Creating data sets with the Code node#
Now that you are familiar with the n8n data structure, you can use it to create your own data sets or simulate node outputs. To do this, use the Code node to write JavaScript code defining your array of objects with the following structure:
1 2 3 4 5 6 7 |
|
For example, the array of objects representing the Ninja turtles would look like this in the Code node:
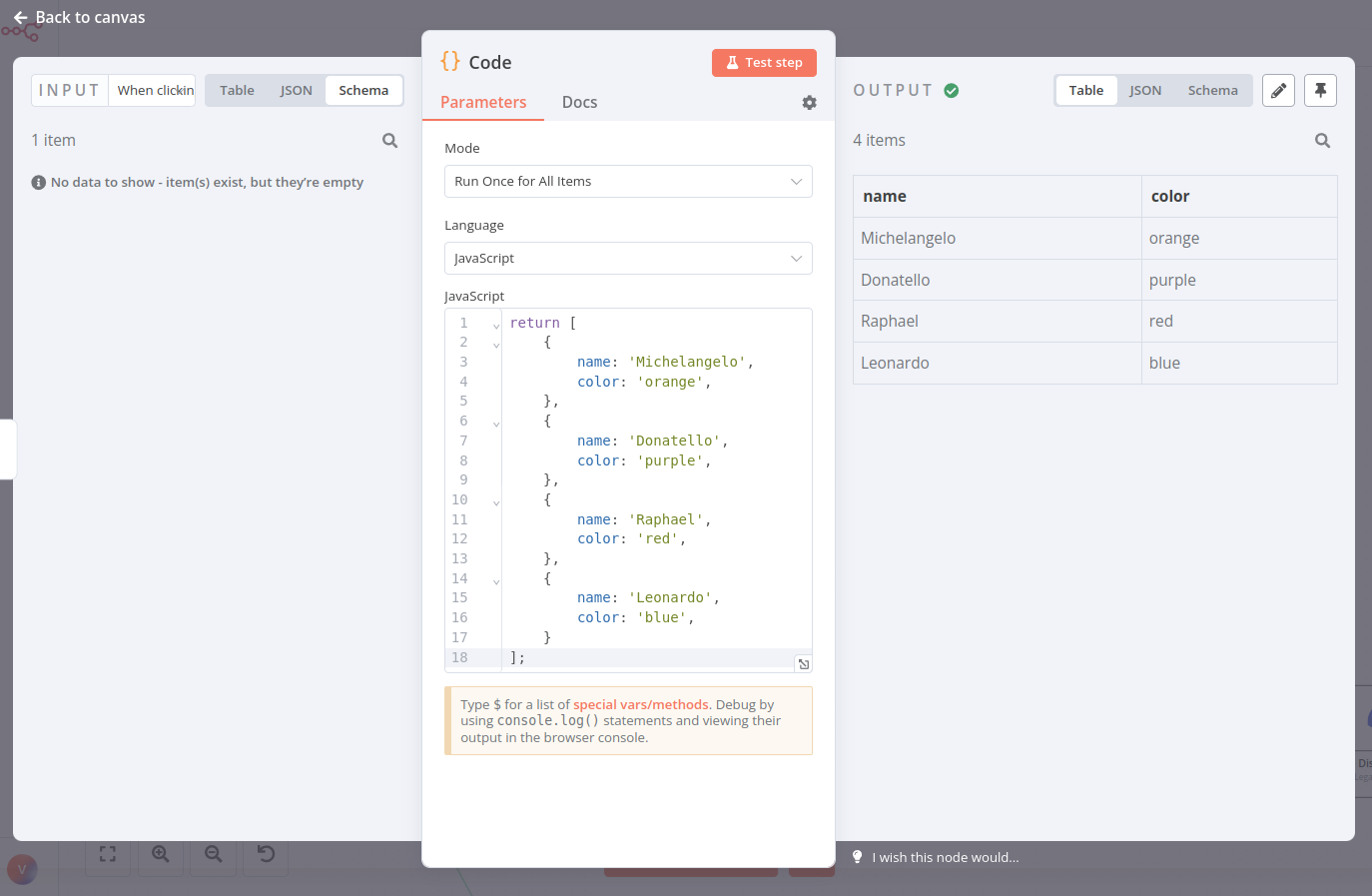
JSON objects
Notice that this array of objects contains an extra key: json
. n8n expects you to wrap each object in an array in another object, with the key json
.
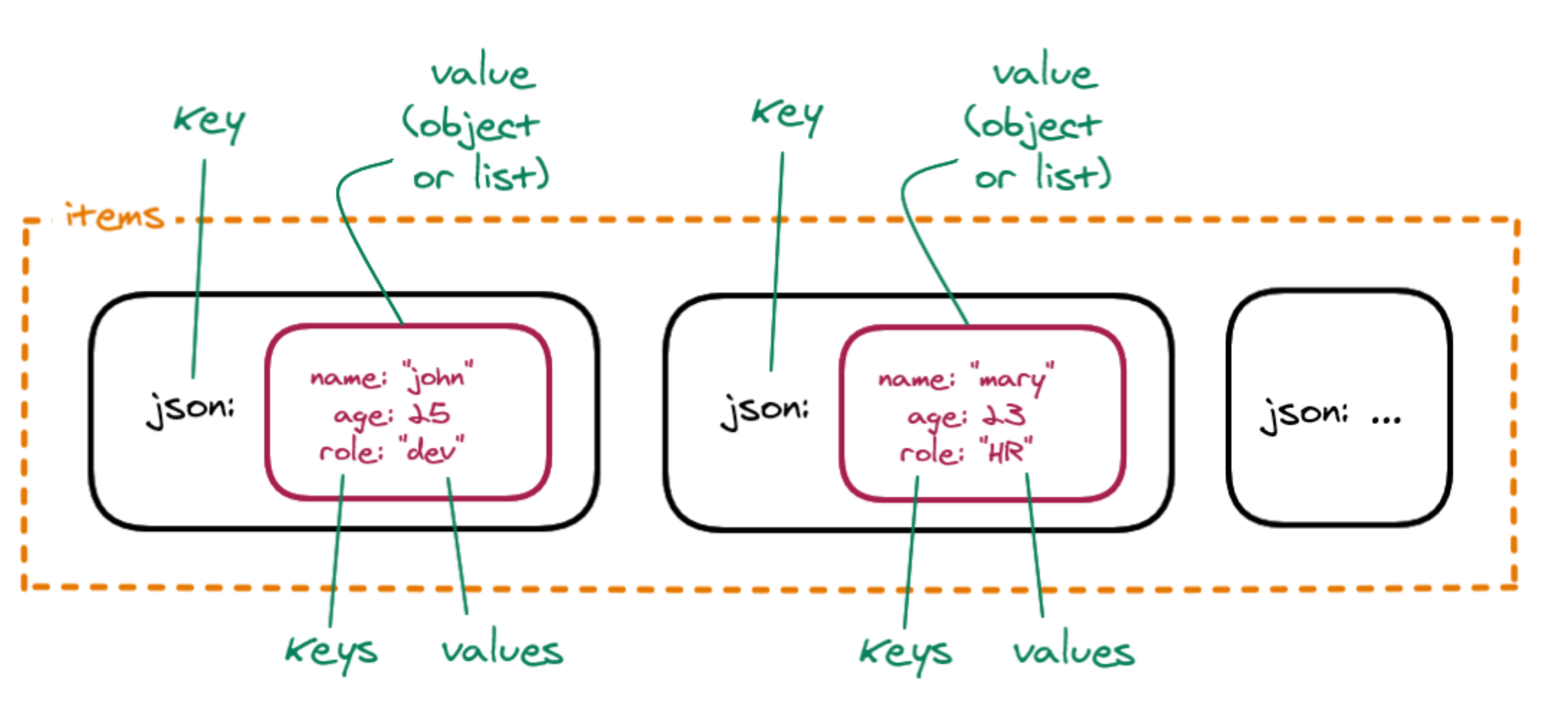
It's good practice to pass the data in the right structure used by n8n. But don't worry if you forget to add the json
key to an item, n8n (version 0.166.0 and above) adds it automatically.
You can also have nested pairs, for example if you want to define a primary and a secondary color. In this case, you need to further wrap the key-value pairs in curly braces {}
.
n8n data structure video
This talk offers a more detailed explanation of data structure in n8n.
Exercise#
In a Code node, create an array of objects named myContacts
that contains the properties name
and email
, and the email
property is further split into personal
and work
.
Show me the solution
In the Code node, in the JavaScript Code field you have to write the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
|
When you execute the Code node, the result should look like this:
Referencing node data with the Code node#
Just like you can use expressions to reference data from other nodes, you can also use some methods and variables in the Code node.
Please make sure you read these pages before continuing to the next exercise.
Exercise#
Let's build on the previous exercise, in which you used the Code node to create a data set of two contacts with their names and emails. Now, connect a second Code node to the first one. In the new node, write code to create a new column named workEmail
that references the work email of the first contact.
Show me the solution
In the Code node, in the JavaScript Code field you have to write the following code:
1 2 3 |
|
When you execute the Code node, the result should look like this:
Transforming data#
The incoming data from some nodes may have a different data structure than the one used in n8n. In this case, you need to transform the data, so that each item can be processed individually.
The two most common operations for data transformation are:
- Creating multiple items from one item
- Creating a single item from multiple items
There are several ways to transform data for the purposes mentioned above:
- Using n8n's data transformation nodes. This is the way to modify the structure of incoming data that contain lists (arrays), without needing to use JavaScript code in the Code node. Use Split Out to separate a single data item containing a list into multiple items, and Aggregate to take separate items, or portions of them, and group them together into individual items.
-
With the Code node, you can write JavaScript functions to modify the data structure of incoming data using the Run Once for All Items mode:
To create multiple items from a single item, you can use this JavaScript code:
1 2 3 4 5
return $input.all().map(item => { return { json: item } });
To create a single item from multiple items, you can use this JavaScript code:
1 2 3 4 5 6 7
return [ { json: { data_object: $input.all().map(item => item.json) } } ];
Exercise#
Use the HTTP Request node to make a GET request to the Quotable API https://api.quotable.io/quotes
. Transform the data in the results
field with the Split Out node and also with the Code node.
Show me the solution
To get the quotes from the Quotable API, execute the HTTP Request node with the following parameters:
- Authentication: None
- Request Method: GET
- URL: https://api.quotable.io/quotes
To transform the data with the Code node, connect this node to the HTTP Request node and write the following code in the JavaScript Code field:
1 2 3 4 5 |
|
To transform the data with the Split Out node, connect this node to the HTTP Request node and set the following parameters:
- Operation: Split Out Items
- Field To Split Out: results
- Include: No Other Fields